mirror of
https://github.com/git/git.git
synced 2024-11-01 23:07:55 +01:00
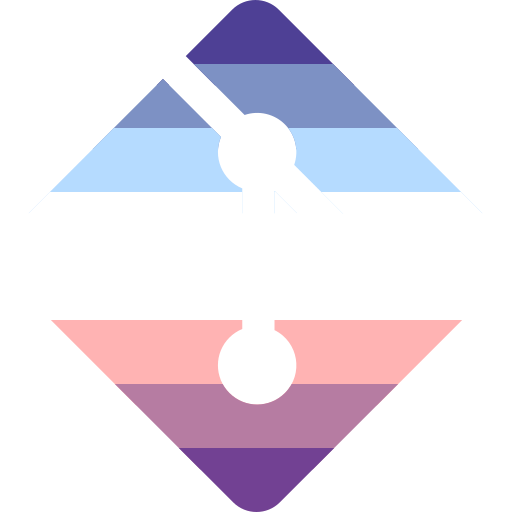
When trying to find a good spot for testing clone with submodules, I got confused where to add a new test file. There are both tests in t560* as well as t57* both testing the clone command. t/README claims the second digit is to indicate the command, which is inconsistent to the current naming structure. Rename all t57* tests to be in t56* to follow the pattern of the digits as laid out in t/README. It would have been less work to rename t56* => t57* because there are less files, but the tests in t56* look more basic and I assumed the higher the last digits the more complicated niche details are tested, so with the patch now it looks more in order to me. Signed-off-by: Stefan Beller <sbeller@google.com> Signed-off-by: Junio C Hamano <gitster@pobox.com>
76 lines
1.9 KiB
Bash
Executable file
76 lines
1.9 KiB
Bash
Executable file
#!/bin/sh
|
|
|
|
test_description='test cloning a repository with detached HEAD'
|
|
. ./test-lib.sh
|
|
|
|
head_is_detached() {
|
|
git --git-dir=$1/.git rev-parse --verify HEAD &&
|
|
test_must_fail git --git-dir=$1/.git symbolic-ref HEAD
|
|
}
|
|
|
|
test_expect_success 'setup' '
|
|
echo one >file &&
|
|
git add file &&
|
|
git commit -m one &&
|
|
echo two >file &&
|
|
git commit -a -m two &&
|
|
git tag two &&
|
|
echo three >file &&
|
|
git commit -a -m three
|
|
'
|
|
|
|
test_expect_success 'clone repo (detached HEAD points to branch)' '
|
|
git checkout master^0 &&
|
|
git clone "file://$PWD" detached-branch
|
|
'
|
|
test_expect_success 'cloned HEAD matches' '
|
|
echo three >expect &&
|
|
git --git-dir=detached-branch/.git log -1 --format=%s >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
test_expect_failure 'cloned HEAD is detached' '
|
|
head_is_detached detached-branch
|
|
'
|
|
|
|
test_expect_success 'clone repo (detached HEAD points to tag)' '
|
|
git checkout two^0 &&
|
|
git clone "file://$PWD" detached-tag
|
|
'
|
|
test_expect_success 'cloned HEAD matches' '
|
|
echo two >expect &&
|
|
git --git-dir=detached-tag/.git log -1 --format=%s >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
test_expect_success 'cloned HEAD is detached' '
|
|
head_is_detached detached-tag
|
|
'
|
|
|
|
test_expect_success 'clone repo (detached HEAD points to history)' '
|
|
git checkout two^ &&
|
|
git clone "file://$PWD" detached-history
|
|
'
|
|
test_expect_success 'cloned HEAD matches' '
|
|
echo one >expect &&
|
|
git --git-dir=detached-history/.git log -1 --format=%s >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
test_expect_success 'cloned HEAD is detached' '
|
|
head_is_detached detached-history
|
|
'
|
|
|
|
test_expect_success 'clone repo (orphan detached HEAD)' '
|
|
git checkout master^0 &&
|
|
echo four >file &&
|
|
git commit -a -m four &&
|
|
git clone "file://$PWD" detached-orphan
|
|
'
|
|
test_expect_success 'cloned HEAD matches' '
|
|
echo four >expect &&
|
|
git --git-dir=detached-orphan/.git log -1 --format=%s >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
test_expect_success 'cloned HEAD is detached' '
|
|
head_is_detached detached-orphan
|
|
'
|
|
|
|
test_done
|