mirror of
https://github.com/git/git.git
synced 2024-10-31 22:37:54 +01:00
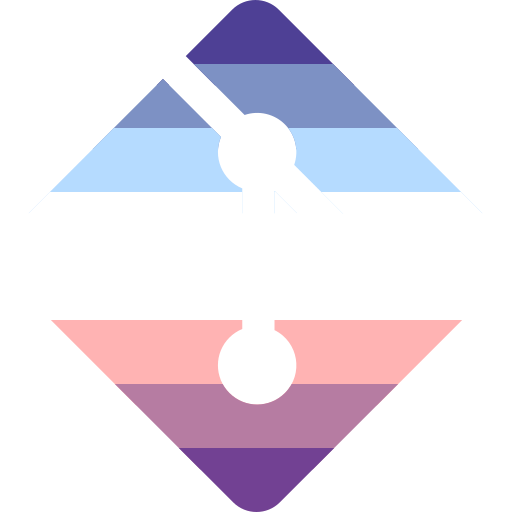
Without this change, gcc -pedantic warns: cache.h: In function 'ce_to_dtype': cache.h:270:21: warning: ISO C forbids braced-groups within expressions [-pedantic] An inline function is more readable anyway. Signed-off-by: Jonathan Nieder <jrnieder@gmail.com> Signed-off-by: Junio C Hamano <gitster@pobox.com>
50 lines
1 KiB
C
50 lines
1 KiB
C
/*
|
|
* Let's make sure we always have a sane definition for ntohl()/htonl().
|
|
* Some libraries define those as a function call, just to perform byte
|
|
* shifting, bringing significant overhead to what should be a simple
|
|
* operation.
|
|
*/
|
|
|
|
/*
|
|
* Default version that the compiler ought to optimize properly with
|
|
* constant values.
|
|
*/
|
|
static inline uint32_t default_swab32(uint32_t val)
|
|
{
|
|
return (((val & 0xff000000) >> 24) |
|
|
((val & 0x00ff0000) >> 8) |
|
|
((val & 0x0000ff00) << 8) |
|
|
((val & 0x000000ff) << 24));
|
|
}
|
|
|
|
#undef bswap32
|
|
|
|
#if defined(__GNUC__) && (defined(__i386__) || defined(__x86_64__))
|
|
|
|
#define bswap32 git_bswap32
|
|
static inline uint32_t git_bswap32(uint32_t x)
|
|
{
|
|
uint32_t result;
|
|
if (__builtin_constant_p(x))
|
|
result = default_swab32(x);
|
|
else
|
|
__asm__("bswap %0" : "=r" (result) : "0" (x));
|
|
return result;
|
|
}
|
|
|
|
#elif defined(_MSC_VER) && (defined(_M_IX86) || defined(_M_X64))
|
|
|
|
#include <stdlib.h>
|
|
|
|
#define bswap32(x) _byteswap_ulong(x)
|
|
|
|
#endif
|
|
|
|
#ifdef bswap32
|
|
|
|
#undef ntohl
|
|
#undef htonl
|
|
#define ntohl(x) bswap32(x)
|
|
#define htonl(x) bswap32(x)
|
|
|
|
#endif
|