mirror of
https://github.com/git/git.git
synced 2024-11-01 23:07:55 +01:00
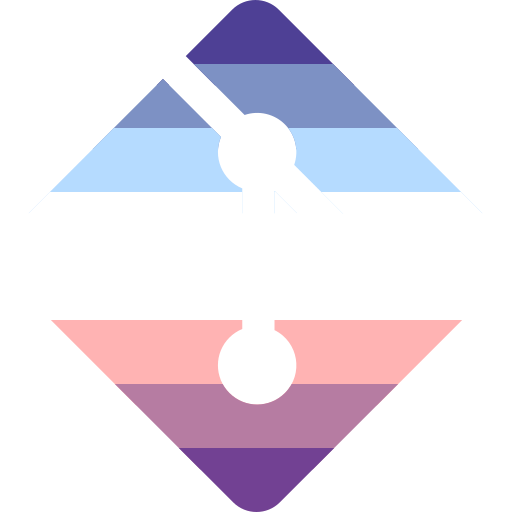
Add a "-u" flag to update the tree as a result of a merge. Right now this code is way too anal about things, and fails merges it shouldn't, but let me fix up the different cases and this will allow for much smoother merging even in the presense of dirty data in the working tree.
60 lines
1.6 KiB
Bash
60 lines
1.6 KiB
Bash
#!/bin/sh
|
|
#
|
|
# Copyright (c) 2005 Linus Torvalds
|
|
#
|
|
# Resolve two trees.
|
|
#
|
|
head="$1"
|
|
merge="$2"
|
|
merge_repo="$3"
|
|
|
|
: ${GIT_DIR=.git}
|
|
: ${GIT_OBJECT_DIRECTORY="${SHA1_FILE_DIRECTORY-"$GIT_DIR/objects"}"}
|
|
|
|
rm -f "$GIT_DIR"/MERGE_HEAD "$GIT_DIR"/ORIG_HEAD
|
|
echo $head > "$GIT_DIR"/ORIG_HEAD
|
|
echo $merge > "$GIT_DIR"/MERGE_HEAD
|
|
|
|
#
|
|
# The remote name is just used for the message,
|
|
# but we do want it.
|
|
#
|
|
if [ "$merge_repo" == "" ]; then
|
|
echo "git-resolve-script <head> <remote> <merge-repo-name>"
|
|
exit 1
|
|
fi
|
|
|
|
common=$(git-merge-base $head $merge)
|
|
if [ -z "$common" ]; then
|
|
echo "Unable to find common commit between" $merge $head
|
|
exit 1
|
|
fi
|
|
|
|
if [ "$common" == "$merge" ]; then
|
|
echo "Already up-to-date. Yeeah!"
|
|
exit 0
|
|
fi
|
|
if [ "$common" == "$head" ]; then
|
|
echo "Updating from $head to $merge."
|
|
echo "Destroying all noncommitted data!"
|
|
echo "Kill me within 3 seconds.."
|
|
sleep 3
|
|
git-read-tree -u -m $head $merge || exit 1
|
|
echo $merge > "$GIT_DIR"/HEAD
|
|
git-diff-tree -p ORIG_HEAD HEAD | git-apply --stat
|
|
exit 0
|
|
fi
|
|
echo "Trying to merge $merge into $head"
|
|
git-read-tree -u -m $common $head $merge || exit 1
|
|
merge_msg="Merge of $merge_repo"
|
|
result_tree=$(git-write-tree 2> /dev/null)
|
|
if [ $? -ne 0 ]; then
|
|
echo "Simple merge failed, trying Automatic merge"
|
|
git-merge-cache git-merge-one-file-script -a
|
|
merge_msg="Automatic merge of $merge_repo"
|
|
result_tree=$(git-write-tree) || exit 1
|
|
fi
|
|
result_commit=$(echo "$merge_msg" | git-commit-tree $result_tree -p $head -p $merge)
|
|
echo "Committed merge $result_commit"
|
|
echo $result_commit > "$GIT_DIR"/HEAD
|
|
git-diff-tree -p ORIG_HEAD HEAD | git-apply --stat
|