mirror of
https://github.com/git/git.git
synced 2024-11-08 18:23:01 +01:00
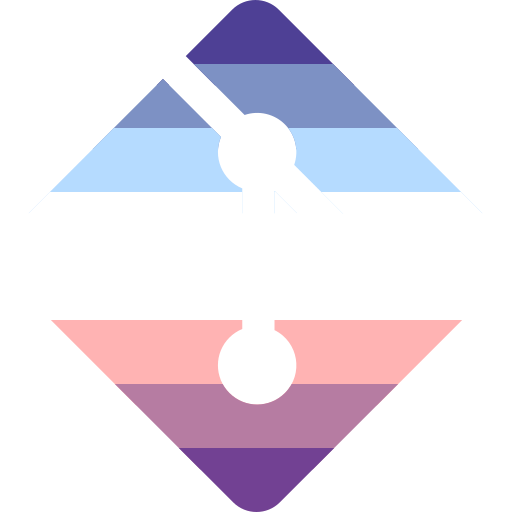
In the man page, there is an example which describes how to remove single commits (although it keeps the changes which were not reverted in the next non-removed commit). Better make sure that it works as expected. Signed-off-by: Johannes Schindelin <johannes.schindelin@gmx.de> Signed-off-by: Junio C Hamano <gitster@pobox.com>
145 lines
3.4 KiB
Bash
Executable file
145 lines
3.4 KiB
Bash
Executable file
#!/bin/sh
|
|
|
|
test_description='git-filter-branch'
|
|
. ./test-lib.sh
|
|
|
|
make_commit () {
|
|
lower=$(echo $1 | tr A-Z a-z)
|
|
echo $lower > $lower
|
|
git add $lower
|
|
test_tick
|
|
git commit -m $1
|
|
git tag $1
|
|
}
|
|
|
|
test_expect_success 'setup' '
|
|
make_commit A
|
|
make_commit B
|
|
git checkout -b branch B
|
|
make_commit D
|
|
make_commit E
|
|
git checkout master
|
|
make_commit C
|
|
git checkout branch
|
|
git merge C
|
|
git tag F
|
|
make_commit G
|
|
make_commit H
|
|
'
|
|
|
|
H=$(git rev-parse H)
|
|
|
|
test_expect_success 'rewrite identically' '
|
|
git-filter-branch H2
|
|
'
|
|
|
|
test_expect_success 'result is really identical' '
|
|
test $H = $(git rev-parse H2)
|
|
'
|
|
|
|
test_expect_success 'rewrite, renaming a specific file' '
|
|
git-filter-branch --tree-filter "mv d doh || :" H3
|
|
'
|
|
|
|
test_expect_success 'test that the file was renamed' '
|
|
test d = $(git show H3:doh)
|
|
'
|
|
|
|
git tag oldD H3~4
|
|
test_expect_success 'rewrite one branch, keeping a side branch' '
|
|
git-filter-branch --tree-filter "mv b boh || :" modD D..oldD
|
|
'
|
|
|
|
test_expect_success 'common ancestor is still common (unchanged)' '
|
|
test "$(git merge-base modD D)" = "$(git rev-parse B)"
|
|
'
|
|
|
|
test_expect_success 'filter subdirectory only' '
|
|
mkdir subdir &&
|
|
touch subdir/new &&
|
|
git add subdir/new &&
|
|
test_tick &&
|
|
git commit -m "subdir" &&
|
|
echo H > a &&
|
|
test_tick &&
|
|
git commit -m "not subdir" a &&
|
|
echo A > subdir/new &&
|
|
test_tick &&
|
|
git commit -m "again subdir" subdir/new &&
|
|
git rm a &&
|
|
test_tick &&
|
|
git commit -m "again not subdir" &&
|
|
git-filter-branch --subdirectory-filter subdir sub
|
|
'
|
|
|
|
test_expect_success 'subdirectory filter result looks okay' '
|
|
test 2 = $(git rev-list sub | wc -l) &&
|
|
git show sub:new &&
|
|
! git show sub:subdir
|
|
'
|
|
|
|
test_expect_success 'setup and filter history that requires --full-history' '
|
|
git checkout master &&
|
|
mkdir subdir &&
|
|
echo A > subdir/new &&
|
|
git add subdir/new &&
|
|
test_tick &&
|
|
git commit -m "subdir on master" subdir/new &&
|
|
git rm a &&
|
|
test_tick &&
|
|
git commit -m "again subdir on master" &&
|
|
git merge branch &&
|
|
git-filter-branch --subdirectory-filter subdir sub-master
|
|
'
|
|
|
|
test_expect_success 'subdirectory filter result looks okay' '
|
|
test 3 = $(git rev-list -1 --parents sub-master | wc -w) &&
|
|
git show sub-master^:new &&
|
|
git show sub-master^2:new &&
|
|
! git show sub:subdir
|
|
'
|
|
|
|
test_expect_success 'use index-filter to move into a subdirectory' '
|
|
git-filter-branch --index-filter \
|
|
"git ls-files -s | sed \"s-\\t-&newsubdir/-\" |
|
|
GIT_INDEX_FILE=\$GIT_INDEX_FILE.new \
|
|
git update-index --index-info &&
|
|
mv \$GIT_INDEX_FILE.new \$GIT_INDEX_FILE" directorymoved &&
|
|
test -z "$(git diff HEAD directorymoved:newsubdir)"'
|
|
|
|
test_expect_success 'author information is preserved' '
|
|
: > i &&
|
|
git add i &&
|
|
test_tick &&
|
|
GIT_AUTHOR_NAME="B V Uips" git commit -m bvuips &&
|
|
git-filter-branch --msg-filter "cat; \
|
|
test \$GIT_COMMIT = $(git rev-parse master) && \
|
|
echo Hallo" \
|
|
preserved-author &&
|
|
test 1 = $(git rev-list --author="B V Uips" preserved-author | wc -l)
|
|
'
|
|
|
|
test_expect_success "remove a certain author's commits" '
|
|
echo i > i &&
|
|
test_tick &&
|
|
git commit -m i i &&
|
|
git-filter-branch --commit-filter "\
|
|
if [ \"\$GIT_AUTHOR_NAME\" = \"B V Uips\" ];\
|
|
then\
|
|
shift;\
|
|
while [ -n \"\$1\" ];\
|
|
do\
|
|
shift;\
|
|
echo \"\$1\";\
|
|
shift;\
|
|
done;\
|
|
else\
|
|
git commit-tree \"\$@\";\
|
|
fi" removed-author &&
|
|
cnt1=$(git rev-list master | wc -l) &&
|
|
cnt2=$(git rev-list removed-author | wc -l) &&
|
|
test $cnt1 -eq $(($cnt2 + 1)) &&
|
|
test 0 = $(git rev-list --author="B V Uips" removed-author | wc -l)
|
|
'
|
|
|
|
test_done
|