mirror of
https://github.com/git/git.git
synced 2024-11-01 06:47:52 +01:00
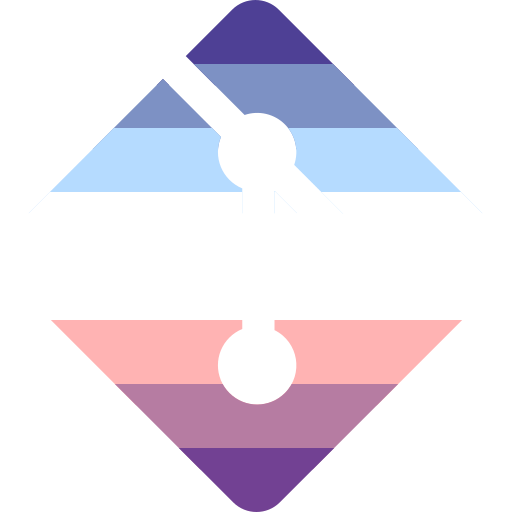
Move the bulk of the code from builtin/mailinfo.c to mailinfo.c so that new callers can start calling mailinfo() directly. Note that a few calls to exit() and die() need to be cleaned up for the API to be truly useful, which will come in later steps. Signed-off-by: Junio C Hamano <gitster@pobox.com>
61 lines
1.6 KiB
C
61 lines
1.6 KiB
C
/*
|
|
* Another stupid program, this one parsing the headers of an
|
|
* email to figure out authorship and subject
|
|
*/
|
|
#include "cache.h"
|
|
#include "builtin.h"
|
|
#include "utf8.h"
|
|
#include "strbuf.h"
|
|
#include "mailinfo.h"
|
|
|
|
static const char mailinfo_usage[] =
|
|
"git mailinfo [-k | -b] [-m | --message-id] [-u | --encoding=<encoding> | -n] [--scissors | --no-scissors] <msg> <patch> < mail >info";
|
|
|
|
int cmd_mailinfo(int argc, const char **argv, const char *prefix)
|
|
{
|
|
const char *def_charset;
|
|
struct mailinfo mi;
|
|
int status;
|
|
|
|
/* NEEDSWORK: might want to do the optional .git/ directory
|
|
* discovery
|
|
*/
|
|
setup_mailinfo(&mi);
|
|
|
|
def_charset = get_commit_output_encoding();
|
|
mi.metainfo_charset = def_charset;
|
|
|
|
while (1 < argc && argv[1][0] == '-') {
|
|
if (!strcmp(argv[1], "-k"))
|
|
mi.keep_subject = 1;
|
|
else if (!strcmp(argv[1], "-b"))
|
|
mi.keep_non_patch_brackets_in_subject = 1;
|
|
else if (!strcmp(argv[1], "-m") || !strcmp(argv[1], "--message-id"))
|
|
mi.add_message_id = 1;
|
|
else if (!strcmp(argv[1], "-u"))
|
|
mi.metainfo_charset = def_charset;
|
|
else if (!strcmp(argv[1], "-n"))
|
|
mi.metainfo_charset = NULL;
|
|
else if (starts_with(argv[1], "--encoding="))
|
|
mi.metainfo_charset = argv[1] + 11;
|
|
else if (!strcmp(argv[1], "--scissors"))
|
|
mi.use_scissors = 1;
|
|
else if (!strcmp(argv[1], "--no-scissors"))
|
|
mi.use_scissors = 0;
|
|
else if (!strcmp(argv[1], "--no-inbody-headers"))
|
|
mi.use_inbody_headers = 0;
|
|
else
|
|
usage(mailinfo_usage);
|
|
argc--; argv++;
|
|
}
|
|
|
|
if (argc != 3)
|
|
usage(mailinfo_usage);
|
|
|
|
mi.input = stdin;
|
|
mi.output = stdout;
|
|
status = !!mailinfo(&mi, argv[1], argv[2]);
|
|
clear_mailinfo(&mi);
|
|
|
|
return status;
|
|
}
|