mirror of
https://github.com/git/git.git
synced 2024-10-31 22:37:54 +01:00
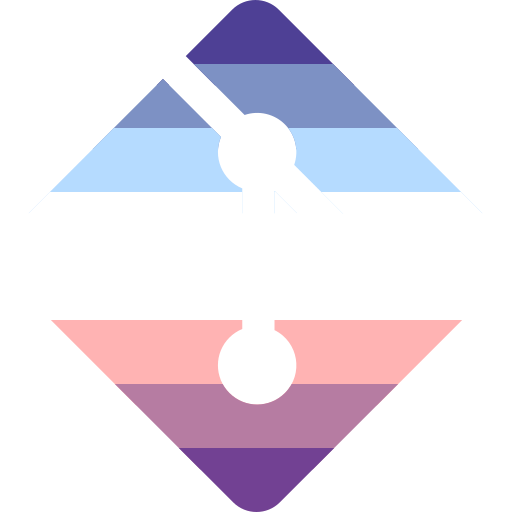
This script uses Documentation/config.txt as input for "git help --config" and "git config" completion but it misses the fact that config.txt includes other txt files. Include all *config.txt as input when scanning for config keys. This could produce false positives, but as long as we stick to the blah-config.txt naming convention, we should be ok. While at there, move diff.* from config.txt to diff-config.txt where all other diff config keys are. Signed-off-by: Nguyễn Thái Ngọc Duy <pclouds@gmail.com> Signed-off-by: Junio C Hamano <gitster@pobox.com>
109 lines
1.6 KiB
Bash
Executable file
109 lines
1.6 KiB
Bash
Executable file
#!/bin/sh
|
|
|
|
die () {
|
|
echo "$@" >&2
|
|
exit 1
|
|
}
|
|
|
|
command_list () {
|
|
grep -v '^#' "$1"
|
|
}
|
|
|
|
get_categories () {
|
|
tr ' ' '\n'|
|
|
grep -v '^$' |
|
|
sort |
|
|
uniq
|
|
}
|
|
|
|
category_list () {
|
|
command_list "$1" |
|
|
cut -c 40- |
|
|
get_categories
|
|
}
|
|
|
|
get_synopsis () {
|
|
sed -n '
|
|
/^NAME/,/'"$1"'/H
|
|
${
|
|
x
|
|
s/.*'"$1"' - \(.*\)/N_("\1")/
|
|
p
|
|
}' "Documentation/$1.txt"
|
|
}
|
|
|
|
define_categories () {
|
|
echo
|
|
echo "/* Command categories */"
|
|
bit=0
|
|
category_list "$1" |
|
|
while read cat
|
|
do
|
|
echo "#define CAT_$cat (1UL << $bit)"
|
|
bit=$(($bit+1))
|
|
done
|
|
test "$bit" -gt 32 && die "Urgh.. too many categories?"
|
|
}
|
|
|
|
define_category_names () {
|
|
echo
|
|
echo "/* Category names */"
|
|
echo "static const char *category_names[] = {"
|
|
bit=0
|
|
category_list "$1" |
|
|
while read cat
|
|
do
|
|
echo " \"$cat\", /* (1UL << $bit) */"
|
|
bit=$(($bit+1))
|
|
done
|
|
echo " NULL"
|
|
echo "};"
|
|
}
|
|
|
|
print_command_list () {
|
|
echo "static struct cmdname_help command_list[] = {"
|
|
|
|
command_list "$1" |
|
|
while read cmd rest
|
|
do
|
|
printf " { \"$cmd\", $(get_synopsis $cmd), 0"
|
|
for cat in $(echo "$rest" | get_categories)
|
|
do
|
|
printf " | CAT_$cat"
|
|
done
|
|
echo " },"
|
|
done
|
|
echo "};"
|
|
}
|
|
|
|
print_config_list () {
|
|
cat <<EOF
|
|
static const char *config_name_list[] = {
|
|
EOF
|
|
grep -h '^[a-zA-Z].*\..*::$' Documentation/*config.txt |
|
|
sed '/deprecated/d; s/::$//; s/, */\n/g' |
|
|
sort |
|
|
while read line
|
|
do
|
|
echo " \"$line\","
|
|
done
|
|
cat <<EOF
|
|
NULL,
|
|
};
|
|
EOF
|
|
}
|
|
|
|
echo "/* Automatically generated by generate-cmdlist.sh */
|
|
struct cmdname_help {
|
|
const char *name;
|
|
const char *help;
|
|
uint32_t category;
|
|
};
|
|
"
|
|
define_categories "$1"
|
|
echo
|
|
define_category_names "$1"
|
|
echo
|
|
print_command_list "$1"
|
|
echo
|
|
print_config_list
|