mirror of
https://github.com/git/git.git
synced 2024-11-01 14:57:52 +01:00
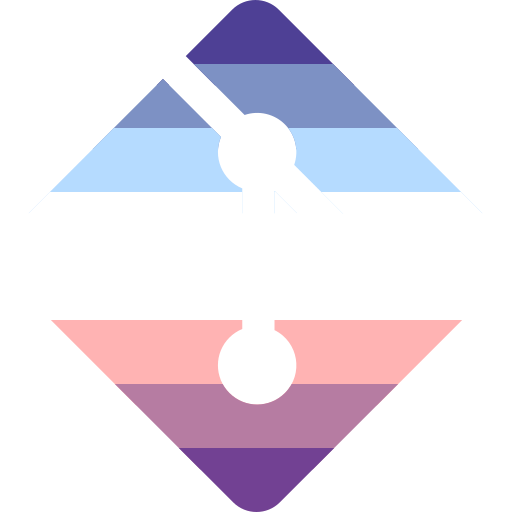
Some SVN repositories contain git repositories within them (hopefully accidentally checked in). Since git refuses to track nested ".git" repositories, this can be a problem when fetching updates from SVN. Thanks to Morgan Christiansson for the report and testing. Signed-off-by: Eric Wong <normalperson@yhbt.net>
101 lines
1.8 KiB
Bash
Executable file
101 lines
1.8 KiB
Bash
Executable file
#!/bin/sh
|
|
#
|
|
# Copyright (c) 2009 Eric Wong
|
|
#
|
|
|
|
test_description='git svn property tests'
|
|
. ./lib-git-svn.sh
|
|
|
|
test_expect_success 'setup repo with a git repo inside it' '
|
|
svn co "$svnrepo" s &&
|
|
(
|
|
cd s &&
|
|
git init &&
|
|
test -f .git/HEAD &&
|
|
> .git/a &&
|
|
echo a > a &&
|
|
svn add .git a &&
|
|
svn commit -m "create a nested git repo" &&
|
|
svn up &&
|
|
echo hi >> .git/a &&
|
|
svn commit -m "modify .git/a" &&
|
|
svn up
|
|
)
|
|
'
|
|
|
|
test_expect_success 'clone an SVN repo containing a git repo' '
|
|
git svn clone "$svnrepo" g &&
|
|
echo a > expect &&
|
|
test_cmp expect g/a
|
|
'
|
|
|
|
test_expect_success 'SVN-side change outside of .git' '
|
|
(
|
|
cd s &&
|
|
echo b >> a &&
|
|
svn commit -m "SVN-side change outside of .git" &&
|
|
svn up &&
|
|
svn log -v | fgrep "SVN-side change outside of .git"
|
|
)
|
|
'
|
|
|
|
test_expect_success 'update git svn-cloned repo' '
|
|
(
|
|
cd g &&
|
|
git svn rebase &&
|
|
echo a > expect &&
|
|
echo b >> expect &&
|
|
test_cmp a expect &&
|
|
rm expect
|
|
)
|
|
'
|
|
|
|
test_expect_success 'SVN-side change inside of .git' '
|
|
(
|
|
cd s &&
|
|
git add a &&
|
|
git commit -m "add a inside an SVN repo" &&
|
|
git log &&
|
|
svn add --force .git &&
|
|
svn commit -m "SVN-side change inside of .git" &&
|
|
svn up &&
|
|
svn log -v | fgrep "SVN-side change inside of .git"
|
|
)
|
|
'
|
|
|
|
test_expect_success 'update git svn-cloned repo' '
|
|
(
|
|
cd g &&
|
|
git svn rebase &&
|
|
echo a > expect &&
|
|
echo b >> expect &&
|
|
test_cmp a expect &&
|
|
rm expect
|
|
)
|
|
'
|
|
|
|
test_expect_success 'SVN-side change in and out of .git' '
|
|
(
|
|
cd s &&
|
|
echo c >> a &&
|
|
git add a &&
|
|
git commit -m "add a inside an SVN repo" &&
|
|
svn commit -m "SVN-side change in and out of .git" &&
|
|
svn up &&
|
|
svn log -v | fgrep "SVN-side change in and out of .git"
|
|
)
|
|
'
|
|
|
|
test_expect_success 'update git svn-cloned repo again' '
|
|
(
|
|
cd g &&
|
|
git svn rebase &&
|
|
echo a > expect &&
|
|
echo b >> expect &&
|
|
echo c >> expect &&
|
|
test_cmp a expect &&
|
|
rm expect
|
|
)
|
|
'
|
|
|
|
test_done
|