mirror of
https://github.com/git/git.git
synced 2024-10-31 22:37:54 +01:00
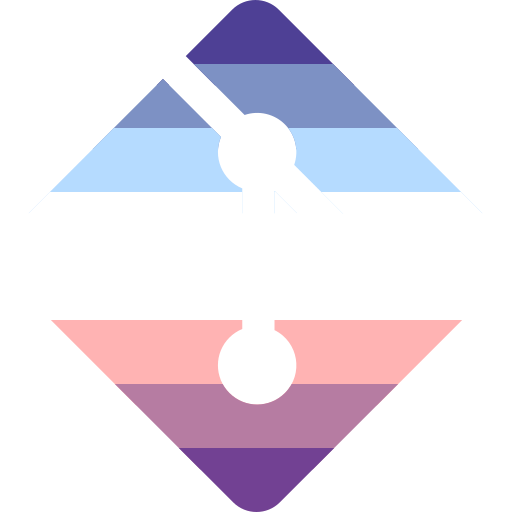
Breaks in a test assertion's && chain can potentially hide failures from earlier commands in the chain by adding " &&" at the end of line to the commands that need them. Signed-off-by: Ramkumar Ramachandra <artagnon@gmail.com> Acked-by: Jonathan Nieder <jrnieder@gmail.com> Signed-off-by: Junio C Hamano <gitster@pobox.com>
109 lines
1.9 KiB
Bash
Executable file
109 lines
1.9 KiB
Bash
Executable file
#!/bin/sh
|
|
|
|
test_description='git rebase - test patch id computation'
|
|
|
|
. ./test-lib.sh
|
|
|
|
test_set_prereq NOT_EXPENSIVE
|
|
test -n "$GIT_PATCHID_TIMING_TESTS" && test_set_prereq EXPENSIVE
|
|
test -x /usr/bin/time && test_set_prereq USR_BIN_TIME
|
|
|
|
count()
|
|
{
|
|
i=0
|
|
while test $i -lt $1
|
|
do
|
|
echo "$i"
|
|
i=$(($i+1))
|
|
done
|
|
}
|
|
|
|
scramble()
|
|
{
|
|
i=0
|
|
while read x
|
|
do
|
|
if test $i -ne 0
|
|
then
|
|
echo "$x"
|
|
fi
|
|
i=$((($i+1) % 10))
|
|
done < "$1" > "$1.new"
|
|
mv -f "$1.new" "$1"
|
|
}
|
|
|
|
run()
|
|
{
|
|
echo \$ "$@"
|
|
/usr/bin/time "$@" >/dev/null
|
|
}
|
|
|
|
test_expect_success 'setup' '
|
|
git commit --allow-empty -m initial &&
|
|
git tag root
|
|
'
|
|
|
|
do_tests()
|
|
{
|
|
pr=$1
|
|
nlines=$2
|
|
|
|
test_expect_success $pr "setup: $nlines lines" "
|
|
rm -f .gitattributes &&
|
|
git checkout -q -f master &&
|
|
git reset --hard root &&
|
|
count $nlines >file &&
|
|
git add file &&
|
|
git commit -q -m initial &&
|
|
git branch -f other &&
|
|
|
|
scramble file &&
|
|
git add file &&
|
|
git commit -q -m 'change big file' &&
|
|
|
|
git checkout -q other &&
|
|
: >newfile &&
|
|
git add newfile &&
|
|
git commit -q -m 'add small file' &&
|
|
|
|
git cherry-pick master >/dev/null 2>&1
|
|
"
|
|
|
|
test_debug "
|
|
run git diff master^\!
|
|
"
|
|
|
|
test_expect_success $pr 'setup attributes' "
|
|
echo 'file binary' >.gitattributes
|
|
"
|
|
|
|
test_debug "
|
|
run git format-patch --stdout master &&
|
|
run git format-patch --stdout --ignore-if-in-upstream master
|
|
"
|
|
|
|
test_expect_success $pr 'detect upstream patch' "
|
|
git checkout -q master &&
|
|
scramble file &&
|
|
git add file &&
|
|
git commit -q -m 'change big file again' &&
|
|
git checkout -q other^{} &&
|
|
git rebase master &&
|
|
test_must_fail test -n \"\$(git rev-list master...HEAD~)\"
|
|
"
|
|
|
|
test_expect_success $pr 'do not drop patch' "
|
|
git branch -f squashed master &&
|
|
git checkout -q -f squashed &&
|
|
git reset -q --soft HEAD~2 &&
|
|
git commit -q -m squashed &&
|
|
git checkout -q other^{} &&
|
|
test_must_fail git rebase squashed &&
|
|
rm -rf .git/rebase-apply
|
|
"
|
|
}
|
|
|
|
do_tests NOT_EXPENSIVE 500
|
|
do_tests EXPENSIVE 50000
|
|
|
|
test_done
|