mirror of
https://github.com/git/git.git
synced 2024-10-31 22:37:54 +01:00
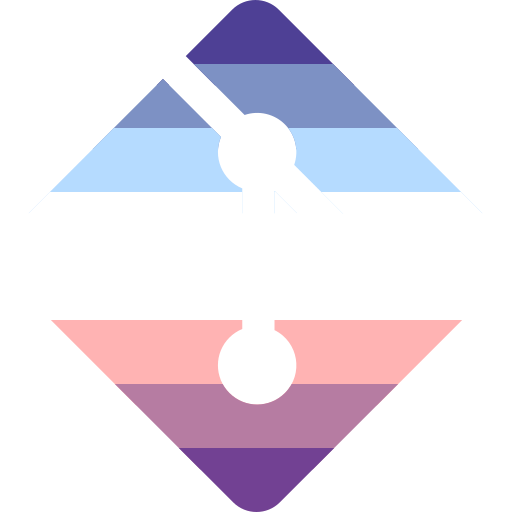
It is a common mistake to call read(2)/pread(2) and forget to anticipate that they may return error with EAGAIN/EINTR when the system call is interrupted. We have xread() helper to relieve callers of read(2) from having to worry about it; add xpread() helper to do the same for pread(2). Update the caller in the builtin/index-pack.c and the mmap emulation in compat/. Signed-off-by: Yiannis Marangos <yiannis.marangos@gmail.com> Signed-off-by: Junio C Hamano <gitster@pobox.com>
40 lines
692 B
C
40 lines
692 B
C
#include "../git-compat-util.h"
|
|
|
|
void *git_mmap(void *start, size_t length, int prot, int flags, int fd, off_t offset)
|
|
{
|
|
size_t n = 0;
|
|
|
|
if (start != NULL || !(flags & MAP_PRIVATE))
|
|
die("Invalid usage of mmap when built with NO_MMAP");
|
|
|
|
start = xmalloc(length);
|
|
if (start == NULL) {
|
|
errno = ENOMEM;
|
|
return MAP_FAILED;
|
|
}
|
|
|
|
while (n < length) {
|
|
ssize_t count = xpread(fd, (char *)start + n, length - n, offset + n);
|
|
|
|
if (count == 0) {
|
|
memset((char *)start+n, 0, length-n);
|
|
break;
|
|
}
|
|
|
|
if (count < 0) {
|
|
free(start);
|
|
errno = EACCES;
|
|
return MAP_FAILED;
|
|
}
|
|
|
|
n += count;
|
|
}
|
|
|
|
return start;
|
|
}
|
|
|
|
int git_munmap(void *start, size_t length)
|
|
{
|
|
free(start);
|
|
return 0;
|
|
}
|