mirror of
https://github.com/git/git.git
synced 2024-10-31 22:37:54 +01:00
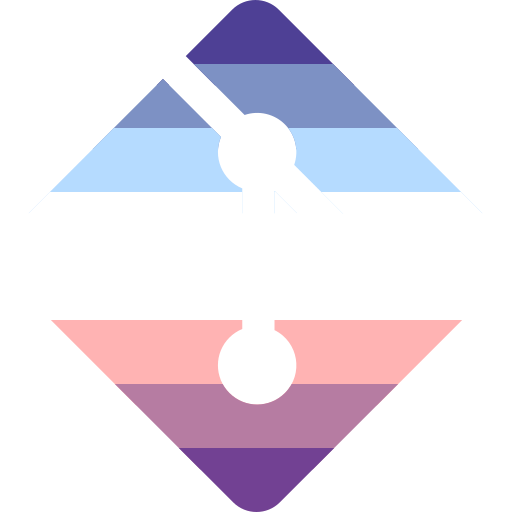
Currently, a branch filter like `--contains`, `--merged`, or `--no-merged` is ignored when we are not in listing mode. For example: git branch --contains=foo bar will create the branch "bar" from the current HEAD, ignoring the `--contains` argument entirely. This is not very helpful. There are two reasonable behaviors for git here: 1. Flag an error; the arguments do not make sense. 2. Implicitly go into `--list` mode This patch chooses the latter, as it is more convenient, and there should not be any ambiguity with attempting to create a branch; using `--contains` and not wanting to list is nonsensical. That leaves the case where an explicit modification option like `-d` is given. We already catch the case where `--list` is given alongside `-d` and flag an error. With this patch, we will also catch the use of `--contains` and other filter options alongside `-d`. Signed-off-by: Jeff King <peff@peff.net> Signed-off-by: Junio C Hamano <gitster@pobox.com>
133 lines
2.2 KiB
Bash
Executable file
133 lines
2.2 KiB
Bash
Executable file
#!/bin/sh
|
|
|
|
test_description='branch --contains <commit>, --merged, and --no-merged'
|
|
|
|
. ./test-lib.sh
|
|
|
|
test_expect_success setup '
|
|
|
|
>file &&
|
|
git add file &&
|
|
test_tick &&
|
|
git commit -m initial &&
|
|
git branch side &&
|
|
|
|
echo 1 >file &&
|
|
test_tick &&
|
|
git commit -a -m "second on master" &&
|
|
|
|
git checkout side &&
|
|
echo 1 >file &&
|
|
test_tick &&
|
|
git commit -a -m "second on side" &&
|
|
|
|
git merge master
|
|
|
|
'
|
|
|
|
test_expect_success 'branch --contains=master' '
|
|
|
|
git branch --contains=master >actual &&
|
|
{
|
|
echo " master" && echo "* side"
|
|
} >expect &&
|
|
test_cmp expect actual
|
|
|
|
'
|
|
|
|
test_expect_success 'branch --contains master' '
|
|
|
|
git branch --contains master >actual &&
|
|
{
|
|
echo " master" && echo "* side"
|
|
} >expect &&
|
|
test_cmp expect actual
|
|
|
|
'
|
|
|
|
test_expect_success 'branch --contains=side' '
|
|
|
|
git branch --contains=side >actual &&
|
|
{
|
|
echo "* side"
|
|
} >expect &&
|
|
test_cmp expect actual
|
|
|
|
'
|
|
|
|
test_expect_success 'branch --contains with pattern implies --list' '
|
|
|
|
git branch --contains=master master >actual &&
|
|
{
|
|
echo " master"
|
|
} >expect &&
|
|
test_cmp expect actual
|
|
|
|
'
|
|
|
|
test_expect_success 'side: branch --merged' '
|
|
|
|
git branch --merged >actual &&
|
|
{
|
|
echo " master" &&
|
|
echo "* side"
|
|
} >expect &&
|
|
test_cmp expect actual
|
|
|
|
'
|
|
|
|
test_expect_success 'branch --merged with pattern implies --list' '
|
|
|
|
git branch --merged=side master >actual &&
|
|
{
|
|
echo " master"
|
|
} >expect &&
|
|
test_cmp expect actual
|
|
|
|
'
|
|
|
|
test_expect_success 'side: branch --no-merged' '
|
|
|
|
git branch --no-merged >actual &&
|
|
>expect &&
|
|
test_cmp expect actual
|
|
|
|
'
|
|
|
|
test_expect_success 'master: branch --merged' '
|
|
|
|
git checkout master &&
|
|
git branch --merged >actual &&
|
|
{
|
|
echo "* master"
|
|
} >expect &&
|
|
test_cmp expect actual
|
|
|
|
'
|
|
|
|
test_expect_success 'master: branch --no-merged' '
|
|
|
|
git branch --no-merged >actual &&
|
|
{
|
|
echo " side"
|
|
} >expect &&
|
|
test_cmp expect actual
|
|
|
|
'
|
|
|
|
test_expect_success 'branch --no-merged with pattern implies --list' '
|
|
|
|
git branch --no-merged=master master >actual &&
|
|
>expect &&
|
|
test_cmp expect actual
|
|
|
|
'
|
|
|
|
test_expect_success 'implicit --list conflicts with modification options' '
|
|
|
|
test_must_fail git branch --contains=master -d &&
|
|
test_must_fail git branch --contains=master -m foo
|
|
|
|
'
|
|
|
|
test_done
|